with that done open up your MainPage.xaml file and we're going to build our UI, pretty straight forward just a button with an image.
<Page
x:Class="pc.Media.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:pc.Media"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<Grid Background="{ThemeResource ApplicationPageBackgroundThemeBrush}">
<StackPanel Orientation="Horizontal">
<Button x:Name="CapturePhoto_BTN" Content="Capture
Photo"/>
<Image x:Name="Image_IMG" Height="900" />
</StackPanel>
</Grid>
</Page>
Ok now let's jump into the codebehind MainPage.xaml.cs, here's where the heavy lifting happens. Firstly what we have to do is create a event handler for our button.
using System;
using Windows.Media.Capture;
using Windows.Storage;
using Windows.UI.Popups;
using Windows.UI.Xaml;
using Windows.UI.Xaml.Controls;
using Windows.UI.Xaml.Media.Imaging;
namespace pc.Media
{
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
this.CapturePhoto_BTN.Click += CapturePhoto_BTN_Click;
}
async void CapturePhoto_BTN_Click(object sender, RoutedEventArgs e)
{
throw new NotImplementedException();
}
}
}
what we did was added the CapturePhoto_BTN_Click event handler to the click event of the CapturePhoto_BTN click event. Now let's add our Photo logic.
notice that we changed our event handler to be asynchronous, with that complete we can now take pictures, the thing to take note of is we're not actually taking the picture, what we're doing is passing off the image capture to the CameraCaptureUI which is taking the image on our behalf and then passes back a StorageFile to our application.
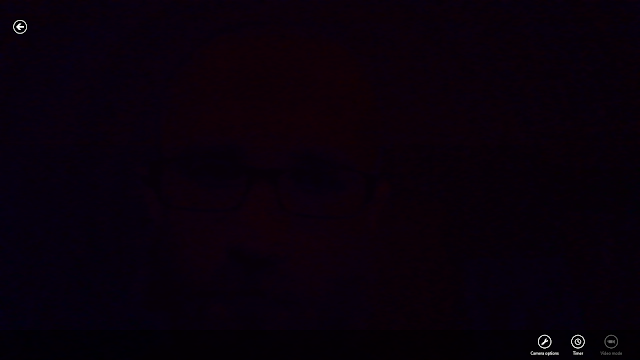
as you see above, this is not our UI, and yes i realize my webcam is broken.
async void CapturePhoto_BTN_Click(object sender, RoutedEventArgs e)
{
var camera = new CameraCaptureUI();
//wait
for the image
StorageFile img = await camera.CaptureFileAsync(CameraCaptureUIMode.Photo);
//Check
if it was sent back
if (img != null)
{
var bitmap = new BitmapImage();
//translate
the stream to an image
bitmap.SetSource(await img.OpenAsync(FileAccessMode.Read));
//set
the UI E
this.Image_IMG.Source = bitmap;
}
else
{
await new MessageDialog("No Photo
recieved").ShowAsync();
}
}
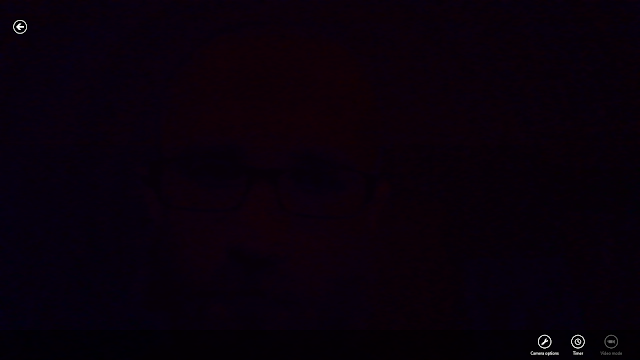
as you see above, this is not our UI, and yes i realize my webcam is broken.